In this article I will show you how you can use powershell to create device collections in bulk using the New-CMDeviceCollection command.
I have built a lot of SCCM environments and one thing I do is create around 200 custom device collections. So I have created a powershell scrip which I use to create these device collections which I will share with you today. I will place the full script I use at the bottom of this article.
To Create SCCM Device Collections via Powershell follow these steps
Step 1 : Create Powershell Script
First we will need to create the powershell script which will hold the variables for the device collections we are going to create.
- Open Windows Powershell ISE
- Copy the below script
# Set Site Code
$SiteCode = Get-PSDrive -PSProvider CMSITE
Set-Location “$($SiteCode.Name):”#Create Software Update Folder
New-Item -Name ‘Software Updates’ -Path “$($SiteCode.Name):\DeviceCollection”#Create Schedule
$ScheduleWeekly = New-CMSchedule -Start “01/01/2017 07:00 PM” -DayOfWeek Sunday -RecurCount 1
The lines 2 to 3 will get the SCCM site code, Do not edit these lines# Set Site Code
$SiteCode = Get-PSDrive -PSProvider CMSITE
Set-Location “$($SiteCode.Name):”The line 6 will create a folder called Software Updates in the root of device collections, Rename Software Updates with the folder name you require#Create Software Update Folder
New-Item -Name ‘Software Updates‘ -Path “$($SiteCode.Name):\DeviceCollection” - The Line 9 will set the variable for the device collection update.
- Replace 07:00 PM with the time you want the update to happen.
- You can also change Sunday with the day you want the update to happen.#Create Schedule
$ScheduleWeekly = New-CMSchedule -Start “01/01/2017 07:00 PM” -DayOfWeek Sunday -RecurCount 1
Step 2 : Create A Device Collection With Direct Membership
To create a device collection with direct membership with no members in it enter the below powershell code.
#Create Device Collection “SU 01 | Disable software Updates”
$NewCollection01 = New-CMDeviceCollection -Name “SU 01 | Disable software Updates” -LimitingCollectionName “All Systems” -RefreshType Both -RefreshSchedule $ScheduleWeekly
Move-CMObject -FolderPath “.\DeviceCollection\Software Updates” -InputObject $NewCollection01
- on Line 12 change “SU 01 | Disable software Updates” to the name you want to give the device collection
- Change “All Systems” to the limiting collection you wish to use
- The Refreshtype is set to both (Incremental and Full updates) or you can set to -RefreshType Periodic (Only Full Updates)
$NewCollection01 = New-CMDeviceCollection -Name “SU 01 | Disable software Updates” -LimitingCollectionName “All Systems” -RefreshType Both -RefreshSchedule $ScheduleWeekly
- The below code will move the device collection to the Software Updates folder, change Software Updates to the folder you require
Move-CMObject -FolderPath “.\DeviceCollection\Software Updates” -InputObject $NewCollection01
Step 3 : Create A Device Collection With Query Membership
To create a device collection with a query membership via powershell use the following command
Add-CMDeviceCollectionQueryMembershipRule -CollectionName “SU 01 | Disable software Updates” -QueryExpression “select SMS_R_System.ResourceId, SMS_R_System.ResourceType, SMS_R_System.Name, SMS_R_System.SMSUniqueIdentifier, SMS_R_System.ResourceDomainORWorkgroup, SMS_R_System.Client from SMS_R_System inner join SMS_G_System_TPM on SMS_G_System_TPM.ResourceID = SMS_R_System.ResourceId” -RuleName “TPM Information”
- Replace “SU 01 | Disable software Updates” with the device collection name you wish to create
- Replace the query with the query you wish to use. Make sure the query goes inside the two “”
- Replace “TPM Information” with the description you wish to give the rule
Add-CMDeviceCollectionQueryMembershipRule -CollectionName “SU 01 | Disable software Updates” -QueryExpression “select SMS_R_System.ResourceId, SMS_R_System.ResourceType, SMS_R_System.Name, SMS_R_System.SMSUniqueIdentifier, SMS_R_System.ResourceDomainORWorkgroup, SMS_R_System.Client from SMS_R_System inner join SMS_G_System_TPM on SMS_G_System_TPM.ResourceID = SMS_R_System.ResourceId” -RuleName “TPM Information“
Step 4 : Bulk Add Devices In To Device Collection
To bulk add devices in to a sccm device collection follow these steps
- Create a .txt file on your machine and add the devices you wish to add to the device collection
- Run the following powershell script replacing C:\Devices.txt with the path to your .txt file and also replace the Collection Name
- Get-Content “C:\Devices.txt” | foreach { Add-CMDeviceCollectionDirectMembershipRule -CollectionName “Collection Name” -ResourceID (Get-CMDevice -Name $_).ResourceID }
Step 5 : Include Device Collection Membership Rule To Device Collection
To add an include device collection membership to a device collection via powershell use the following code
Add-CMDeviceCollectionIncludeMembershipRule -CollectionName “SU 01 | Disable software Updates” -IncludeCollectionName “All Windows 10 Devices”
- Replace “SU 01 | Disable software Updates” with the collection you wish to update.
- Replace “All Windows 10 Devices” with the device collection you want to include.
Add-CMDeviceCollectionIncludeMembershipRule -CollectionName “SU 01 | Disable software Updates” -IncludeCollectionName “All Windows 10 Devices“
Step 6 : Exclude Device Collection Membership Rule To Device Collection
To exclude device collection membership to a device collection via powershell use the following code
Add-CMDeviceCollectionExcludeMembershipRule -CollectionName “SU 01 | Disable software Updates” -excludeCollectionName “All Windows 2012 Devices”
- Replace “SU 01 | Disable software Updates” with the collection you wish to update.
- Replace “All Windows 2012 Devices” with the device collection you want to exclude.
Add-CMDeviceCollectionExcludeMembershipRule -CollectionName “SU 01 | Disable software Updates” -excludeCollectionName “All Windows 2012 Devices“
Full Script Used
Below is the script that I currently use. Feel free to copy and use it. If you have any questions about anything in this article please post about it in a comment below
# Set Site Code
$SiteCode = Get-PSDrive -PSProvider CMSITE
Set-Location “$($SiteCode.Name):”
#Create Software Update Folder
New-Item -Name ‘Software Updates’ -Path “$($SiteCode.Name):\DeviceCollection”
#Create Schedule
$ScheduleWeekly = New-CMSchedule -Start “01/01/2017 07:00 PM” -DayOfWeek Sunday -RecurCount 1
$ScheduleWeekly2 = New-CMSchedule -Start “01/01/2017 07:00 PM” -DayOfWeek Monday -RecurCount 1
#Create Device Collection With Direct Membership “SU 01 | Disable software Updates”
$NewCollection01 = New-CMDeviceCollection -Name “SU 01 | Disable software Updates” -LimitingCollectionName “All Systems” -RefreshType Both -RefreshSchedule $ScheduleWeekly
Move-CMObject -FolderPath “.\DeviceCollection\Software Updates” -InputObject $NewCollection01
Get-Content “C:\Devices.txt” | foreach { Add-CMDeviceCollectionDirectMembershipRule -CollectionName “Collection Name” -ResourceID (Get-CMDevice -Name $_).ResourceID }
#Create Device Collection With Query Membership “SU 01 | Disable software Updates Query”
$NewCollection02 = Add-CMDeviceCollectionQueryMembershipRule -CollectionName “SU 01 | Disable software Updates Query” -QueryExpression “select SMS_R_System.ResourceId, SMS_R_System.ResourceType, SMS_R_System.Name, SMS_R_System.SMSUniqueIdentifier, SMS_R_System.ResourceDomainORWorkgroup, SMS_R_System.Client from SMS_R_System inner join SMS_G_System_TPM on SMS_G_System_TPM.ResourceID = SMS_R_System.ResourceId” -RuleName “TPM Information”
Move-CMObject -FolderPath “.\DeviceCollection\Software Updates” -InputObject $NewCollection02
#Include Device Collection Membership Rule To Device Collection
Add-CMDeviceCollectionIncludeMembershipRule -CollectionName “SU 01 | Disable software Updates” -IncludeCollectionName “All Windows 10 Devices”
#Exclude Device Collection Membership Rule To Device Collection
Add-CMDeviceCollectionExcludeMembershipRule -CollectionName “SU 01 | Disable software Updates” -excludeCollectionName “All Windows 2012 Devices”
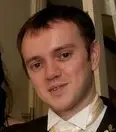
Hello, I am the owner of this site. I have 25+ years experience of IT. Check us out on the below social platforms.